Create WordPress Posts from an API
It is relatively easy to automate post creation in WordPress while pulling data from an api, and to add some pieces of data into custom fields. First, you need to grab some remote data. In this example we’ll get some data from Reddit, specifically the WordPress subreddit – https://reddit.com/r/WordPress
Query Reddit’s API
First, lets get some data from Reddit. We can use the wp_remote_get() function here to get data from Reddit’s REST API endpoint – https://www.reddit.com/r/Wordpress/.json. This endpoint uses the same pagination as a page on Reddit, so you will only get the data for 25 posts at a time.
1 2 3 4 5 6 7 8 9 |
$reddit_data = wp_remote_get( 'https://www.reddit.com/r/Wordpress/.json' ); $reddit_data_decode = json_decode( $reddit_data['body'] ); foreach ( $reddit_data_decode->data->children as $item ) { $post_title = $item->data->title; // post title $reddit_author = $item->data->author; // author $up_votes = $item->data->ups; // up votes } |
After first retrieving the data, we need to decode the JSON into a PHP object. We only need the body
of the response so we reference that. From there we can get individual items from the array as we would any other PHP object, so here we loop through all of the data to get the title, Reddit author, and number of ‘up votes’ for each post.
Lets Create some Posts
Next, we need to do something with this data. We can use the WordPress function wp_insert_post() to create our posts.
1 2 3 4 5 6 7 8 9 10 11 12 |
foreach ( $reddit_data_decode->data->children as $item ) { $post_title = $item->data->title; // post title $reddit_author = $item->data->author; // author $up_votes = $item->data->ups; // up votes $my_post = array( 'post_title' => $post_title, 'post_status' => 'publish', 'post_type' => 'post', ); $post_id = wp_insert_post( $my_post ); |
If we run this code we should wind up with 25 posts (with only a title so far) that match the 25 newest posts on the /r/WordPress.
Finally, Add some Custom Fields
Using the Advanced Custom Fields Pro plugin I’ve created a field group for posts with custom fields for Reddit Author
and Up Votes
. We can use an ACF function here to add the custom field data to our new posts. We just need to add a few more lines to our code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
foreach ( $reddit_data_decode->data->children as $item ) { $post_title = $item->data->title; // post title $reddit_author = $item->data->author; // author $up_votes = $item->data->ups; // up votes $my_post = array( 'post_title' => $post_title, 'post_status' => 'publish', 'post_type' => 'post', ); $post_id = wp_insert_post( $my_post ); update_field( 'reddit_author', $reddit_author, $post_id ); update_field( 'up_votes', $up_votes, $post_id ); |
We are already returning the post ID of each newly created post when we use the wp_insert_post
function, so all we need to do is pass this ID into the update_field() function.
If we run this code, we will get our posts complete with some custom field data.
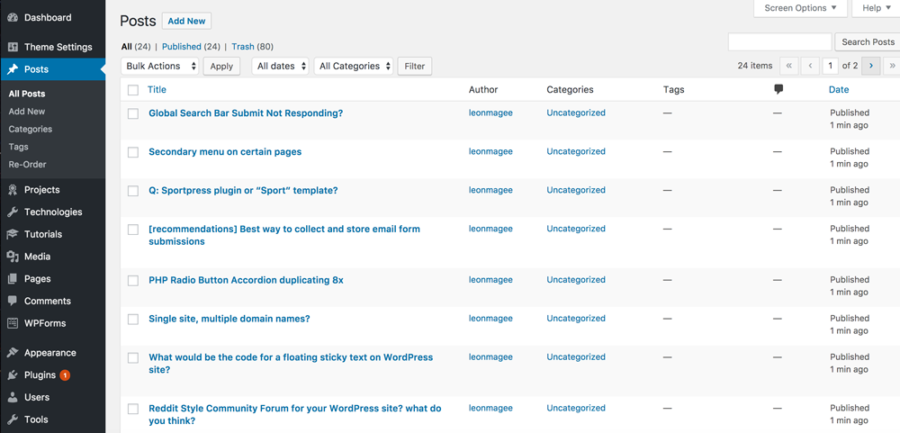
Now that you have your posts created, you can choose to output and style this data any way you want. While this may seem very simple, it’s really powerful since you can do the same thing with any API you have access to, and you can pull in an unlimited number of pieces of data into custom fields or else use the data however you choose.